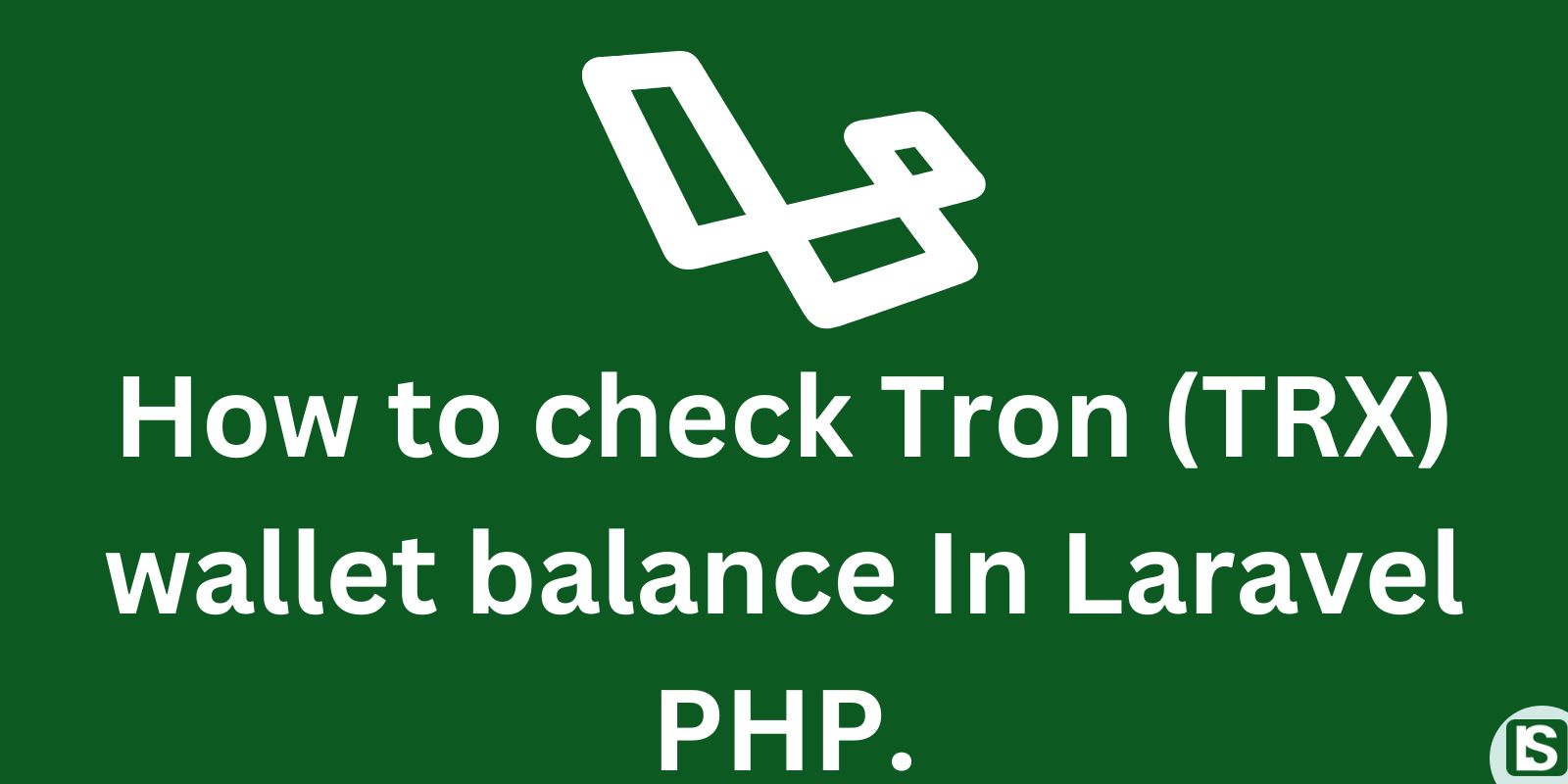
Table Of Contents
Introduction
This article will guide you through the process of retrieving the TRX balance of a Tron wallet address using the PHP Laravel Framework. We will utilise the Trongrid node infrastructure to establish a connection with the Tron blockchain.
prerequisites
Begin by setting up a Laravel application. We'll leverage the Guzzle HTTP package , which is pre-installed with Laravel. Additionally, you'll require a Trongrid API key for connecting to the Tron main net, obtainable from the Trongrid Website .
Get wallet TRX balance
To fetch the TRX balance of the wallet, we'll send an HTTP POST request to Trongrid's wallet/getaccount endpoint, along with the wallet address.
<?php
namespace App\Http\Controllers;
use GuzzleHttp\Client;
use Illuminate\Http\Request;
class TronController extends Controller
{
public function checkTrxBalance($address = 'TPHvvgrPxywW2YPDzhcdeBpGemdR3a9eSd'){
$client = new Client();
$body = "{\"address\":\"$address\",\"visible\":true}";
$response = $client->request('POST', 'https://api.trongrid.io/wallet/getaccount', [
'body' => $body,
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
'TRON-PRO-API-KEY' => '' //Your trongrid api key
],
]);
$result = json_decode($response->getBody()); //change the json data to object
$balance = $result->balance; // get the balance in sun
$trx_balance = $balance / 1000000; // convert the balance to TRX
return $trx_balance;
}
}
The checkTrxBalance() function in the snippet above is invoked with the $address parameter, and it effectively returns the TRX balance of the specified wallet address.
Conclusion
You've acquired the skill of retrieving the tron wallet TRX balance with PHP leveraging the Trongrid infrastructure, thereby enhancing your proficiency in integrating crypto payments within Laravel.
Comment
Login to comment