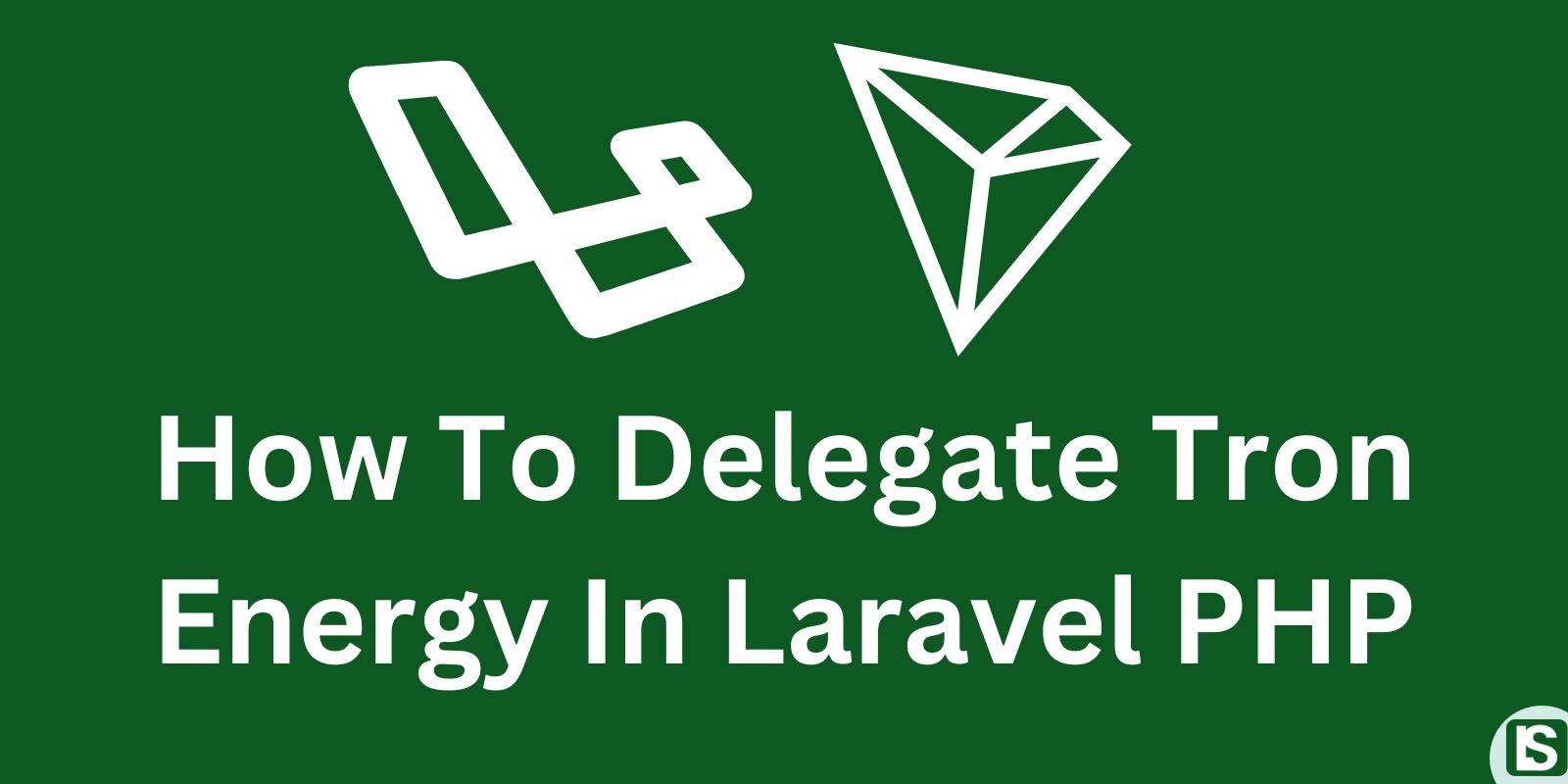
Are you building a TRON-integrated app with Laravel PHP and want to reduce fees on TRC20 token transactions? Delegating TRON energy from one wallet to another is a cost-saving and scalable solution.
In this guide, you’ll learn exactly how to:
- Check delegatable energy using the TRON API
- Calculate the TRX equivalent of energy (required in sun units)
- Delegate energy to another wallet
- Sign and broadcast the transaction securely using a PHP-based signer
This article is built entirely around a real-world Laravel controller, using Guzzle HTTP for API requests and a php tron signer.
🔗 Table of Contents
🚀 Why Delegate TRON Energy?
On the TRON blockchain:
- Energy is used to execute smart contracts (e.g. TRC20 transfers).
- You can freeze TRX to generate energy and delegate it to other wallets.
- Delegation improves UX by allowing users to transact gas-free.
🛠️ Requirements
- Laravel 8 or higher
- Guzzle HTTP client
- TRON wallet with delegatable energy
- TronGrid API Key
- LakeScripts PHP TRON Transaction Signer
.env
TRONGRID_API_KEY=your_api_key
TRONGRID_URI=https://api.trongrid.io
🧠 Laravel Controller: TRON Energy Delegation
1️⃣ Controller Setup
protected $trongrid_uri;
protected $trongrid_api_key;
public function __construct(array $attributes = [])
{
parent::__construct($attributes);
$this->trongrid_api_key = getenv('TRONGRID_API_KEY');
$this->trongrid_uri = getenv('TRONGRID_URI');
}
2️⃣ Check Delegatable Energy
This function checks if the sender wallet has delegatable energy.
public function checkEnergy($address)
{
$client = new Client();
$body = "{\"owner_address\":\"$address\",\"type\":1,\"visible\":true}";
$response = $client->post($this->trongrid_uri . '/wallet/getcandelegatedmaxsize', [
'body' => $body,
'headers' => ['accept' => 'application/json', 'content-type' => 'application/json'],
]);
return json_decode($response->getBody());
}
3️⃣ Get Sender’s Energy Stats
This gives you the account's energy stats including total limit and total weight. These are crucial for calculating energy-to-TRX equivalence.
public function getAccountResources($address)
{
$client = new Client();
$body = "{\"address\":\"$address\",\"visible\":true}";
$response = $client->post($this->trongrid_uri . '/wallet/getaccountresource', [
'body' => $body,
'headers' => ['accept' => 'application/json', 'content-type' => 'application/json'],
]);
return json_decode($response->getBody());
}
4️⃣ Delegate Resource (in sun)
public function delegate($sender, $receiver, $amount)
{
$client = new Client();
$body = "{\"owner_address\":\"$sender\",\"receiver_address\":\"$receiver\",\"balance\":$amount,\"resource\":\"ENERGY\",\"lock\":false,\"visible\":true}";
$response = $client->post($this->trongrid_uri . '/wallet/delegateresource', [
'body' => $body,
'headers' => ['accept' => 'application/json', 'content-type' => 'application/json'],
]);
return json_decode($response->getBody());
}
5️⃣ Broadcast Signed Transaction
After signing, you attach the signature to the transaction and broadcast it to the TRON network.
public function broadcastTransaction($transaction)
{
$client = new Client();
$body = json_encode($transaction);
$response = $client->post($this->trongrid_uri . '/wallet/broadcasttransaction', [
'body' => $body,
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
'TRON-PRO-API-KEY' => $this->trongrid_api_key,
],
]);
return json_decode($response->getBody());
}
6️⃣ Sign the Transaction
Once you get the txID
from a successful delegation, you need to sign it using the sender’s private key.
$signature = $this->signTrx($delegation->txID, $sender_private_key);
✅ You’ll need a secure TRON transaction signing algorithm from LakeScripts PHP TRON Signer.
public function signTrx($transaction_id, $private_key)
{
// Use LakeScripts TRON PHP Signer for secure signing
// https://lakescripts.com/shop/php-tron-signer---sign-tron-blockchain-transactions-in-your-php-application
}
🧪 Final Delegation Method
public function delegateEnergy($sender_address, $sender_private_key, $receiver_address, $required_energy)
{
$delegatable = $this->checkEnergy($sender_address);
if (isset($delegatable->max_size)) {
$delegatable_trx = $delegatable->max_size / 1_000_000;
$resources = $this->getAccountResources($sender_address);
$total_limit = $resources->TotalEnergyLimit;
$total_weight = $resources->TotalEnergyWeight;
$trx_equivalent = ($required_energy * $total_weight) / $total_limit;
$sun_equivalent = $trx_equivalent * 1_000_000;
if ($delegatable_trx > $trx_equivalent) {
$delegation = $this->delegate($sender_address, $receiver_address, $sun_equivalent);
if (isset($delegation->txID) && $delegation->visible) {
$signature = $this->signTrx($delegation->txID, $sender_private_key);
$delegation->signature = [$signature];
return $this->broadcastTransaction($delegation);
}
}
}
return false;
}
🧠 Energy-to-TRX Conversion Explained
The /wallet/delegateresource
endpoint expects TRX value in sun (1 TRX = 1,000,000 sun), not energy units. To convert:
$trx_equivalent = ($required_energy * $total_weight) / $total_limit;
$sun_equivalent = $trx_equivalent * 1000000;
✅ Final Thoughts
Delegating TRON energy in Laravel gives you the flexibility to programmatically support other wallets and improve the efficiency of your blockchain applications. Whether you're building a TRC20 token platform, a smart contract-based dApp, or a blockchain-powered game, delegating energy allows you to abstract gas costs away from the user, offering a smoother Web3 experience.
With the code and method provided in this tutorial, you can:
- Check a wallet’s available delegation capacity
- Compute energy-to-TRX equivalent accurately
- Sign transactions securely with PHP using an offline signer
- Delegate and broadcast TRON energy like a pro
If you're ready to take it further, consider automating energy delegation as a service within your Laravel app or building a delegation pool for TRC20 users.
For secure transaction signing, check out the LakeScripts PHP TRON signer for private-key-safe signing.
Comment
Login to comment