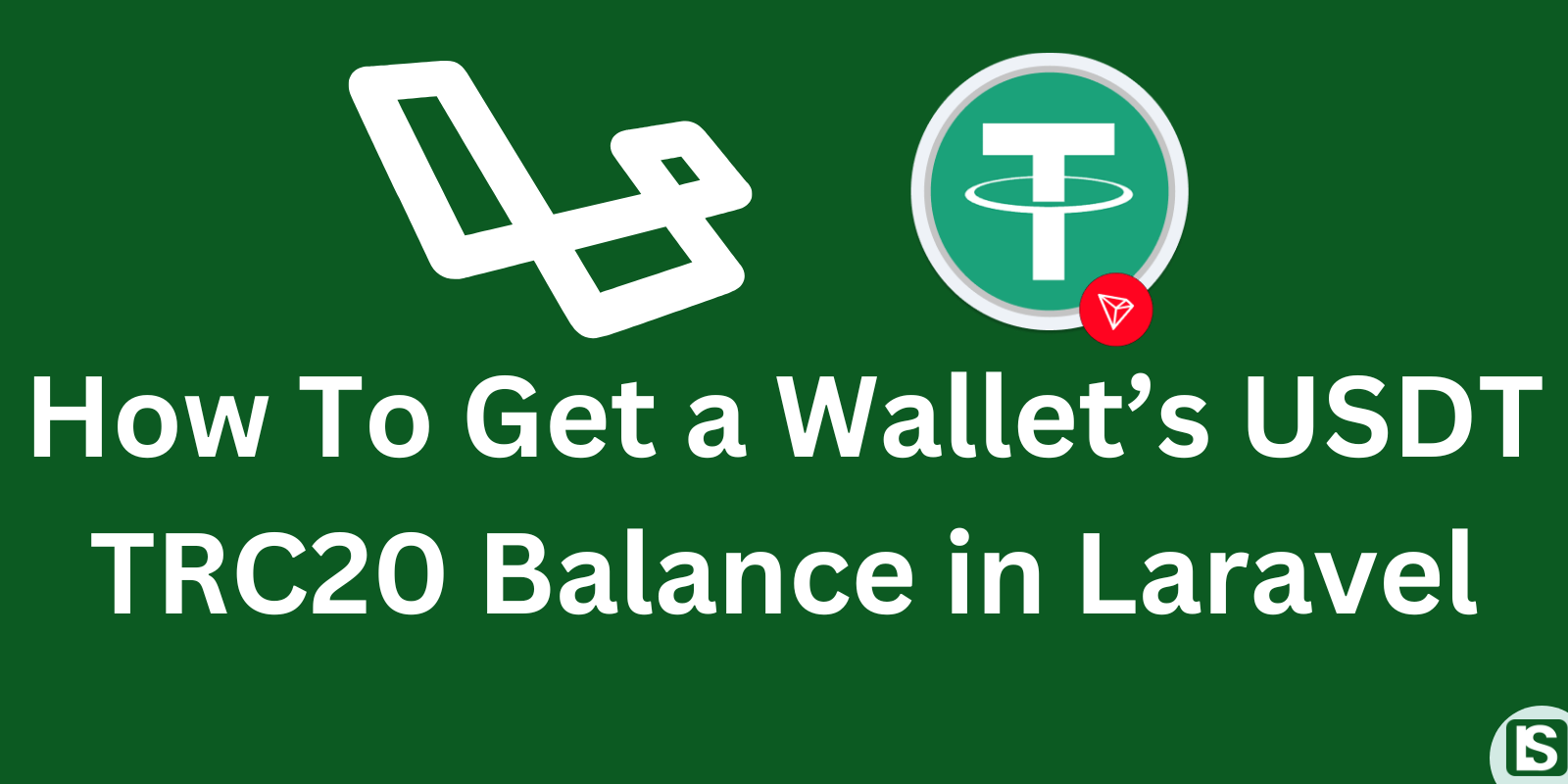
Table Of Contents
- Why USDT TRC20?
- Step-by-Step: How to Get USDT TRC20 Balance in Laravel
- Required Elements
- Notes
- Conclusion
π Why USDT TRC20?
USDT on the TRON network (TRC20) is a popular stablecoin because of its low transaction fees and fast confirmation times. It's widely used in decentralized apps (DApps), wallets, and exchanges. For Laravel developers, integrating with the TRON network to fetch USDT balances can enhance crypto wallet functionality and enable smoother user experiences.
π Step-by-Step: How to Get USDT TRC20 Balance in Laravel
β 1. Set Up Guzzle HTTP Client
Ensure you have Guzzle installed. Itβs a powerful HTTP client used to make requests to external APIs.
composer require guzzlehttp/guzzle
β 2. Create the TronController
Hereβs a full example of how to get a TRC20 wallet balance for USDT using Laravel:
<?php
namespace App\Http\Controllers;
use GuzzleHttp\Client;
class TronController extends Controller
{
public function getUsdtTrc20($address_hex)
{
$owner = 'THPvaUhoh2Qn2y9THCZML3H815hhFhn5YC';
$contract_address = 'TR7NHqjeKQxGTCi8q8ZY4pL8otSzgjLj6t';
$new_address_hex = substr($address_hex, 2);
$padded_address_hex = str_pad($new_address_hex, 64, 0, STR_PAD_LEFT);
$client = new Client();
$body = json_encode([
'owner_address' => $owner,
'contract_address' => $contract_address,
'function_selector' => 'balanceOf(address)',
'parameter' => $padded_address_hex,
'visible' => true,
]);
$response = $client->request(
'POST',
'https://api.trongrid.io/wallet/triggerconstantcontract',
[
'body' => $body,
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
'TRON-PRO-API-KEY' => env('TRON_API_KEY'),
],
]
);
$result = json_decode($response->getBody());
$balance_hex = $result->constant_result[0];
if ($balance_hex == '0000000000000000000000000000000000000000000000000000000000000000') {
return 0;
} else {
$trimed = ltrim($balance_hex, 0);
$amount_numeric = gmp_strval(gmp_init($trimed, 16), 10);
return $amount_numeric / 1000000;
}
}
}
π Required Elements
- TronGrid API Key : Get your API key from TronGrid .
-
USDT Contract Address
: Use
TR7NHqjeKQxGTCi8q8ZY4pL8otSzgjLj6t
. - GMP PHP Extension : Ensure it's enabled to handle large hexadecimal values.
π‘ Notes
- TRON addresses passed into the function must be in hex format .
-
USDT values use 6 decimal places, so divide the raw number by
1000000
. - This method works for any TRC20 token β just change the contract address.
β Conclusion
Fetching the USDT TRC20 wallet balance in Laravel is straightforward with the right tools and setup. By integrating the TronGrid API and handling the TRC20 contract logic, you can easily empower your Laravel app with blockchain functionality.
Want to expand this to send TRC20 tokens or work with other smart contracts? Reach outβIβm here to help!
Comment
Login to comment