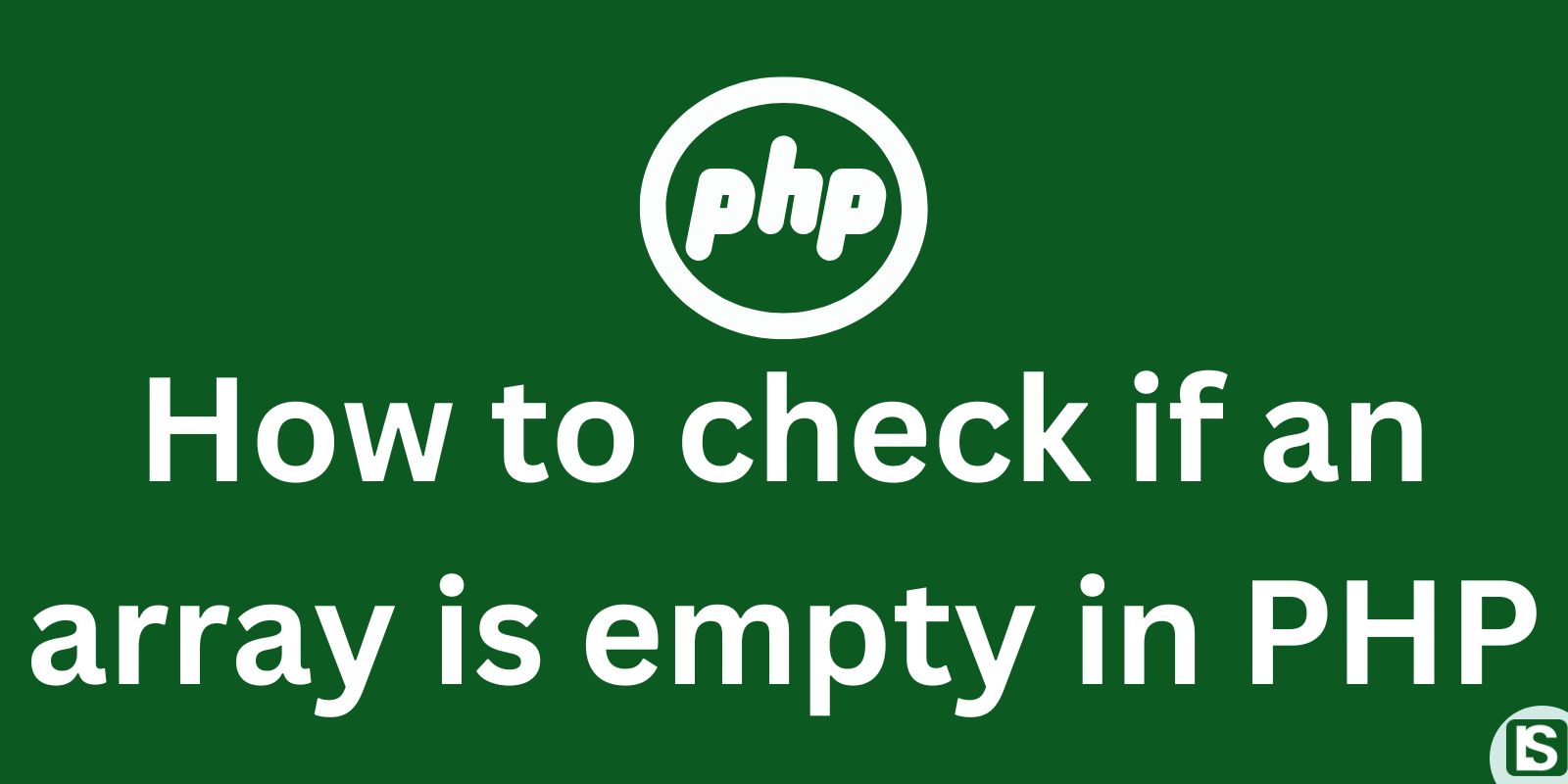
Table Of Contents
- Introduction
- 1. Using the empty() function
- 2. Using the count() function
- 3. Using the === [] expression
- Conclusion
Introduction
As a PHP Developer, I frequently encounter the task of verifying if an array is empty while writing PHP code. In this article, we'll delve into several effective methods for checking the emptiness of a PHP array.
1. Using the empty() function
My most favourite and straightforward way of checking if a PHP array is empty is by using the empty() function. This is a PHP built-in function used for checking if a variable is empty and it does so for arrays too.
If the array is empty, the function will return true
$cars = [];
$is_empty = empty($cars); // true
If the array is not empty, the function will return false.
$cars = ['subaru', 'jeep', 'ford'];
$is_empty = empty($cars); // false
2. Using the count() function
The count() function counts the number of elements in an array and it returns 0 if the array is empty.
$cars = [];
if(count($cars) == 0){
// array is empty
}else{
// array is not empty
}
3. Using the === [] expression
This simple expression also checks if the variable is an empty array and not an integer or a string.
The expression returns true if the array is empty.
$cars = [];
$is_empty = $cars === []; // true
The expression returns false if the array is not empty
$cars = ['subaru', 'jeep', 'ford'];
$is_empty = $cars === []; // false
Conclusion
In this article, we have explored various ways of checking if a PHP array is empty, including the use of the empty() function, the count() function and the === [ ] expression
Comment
Login to comment