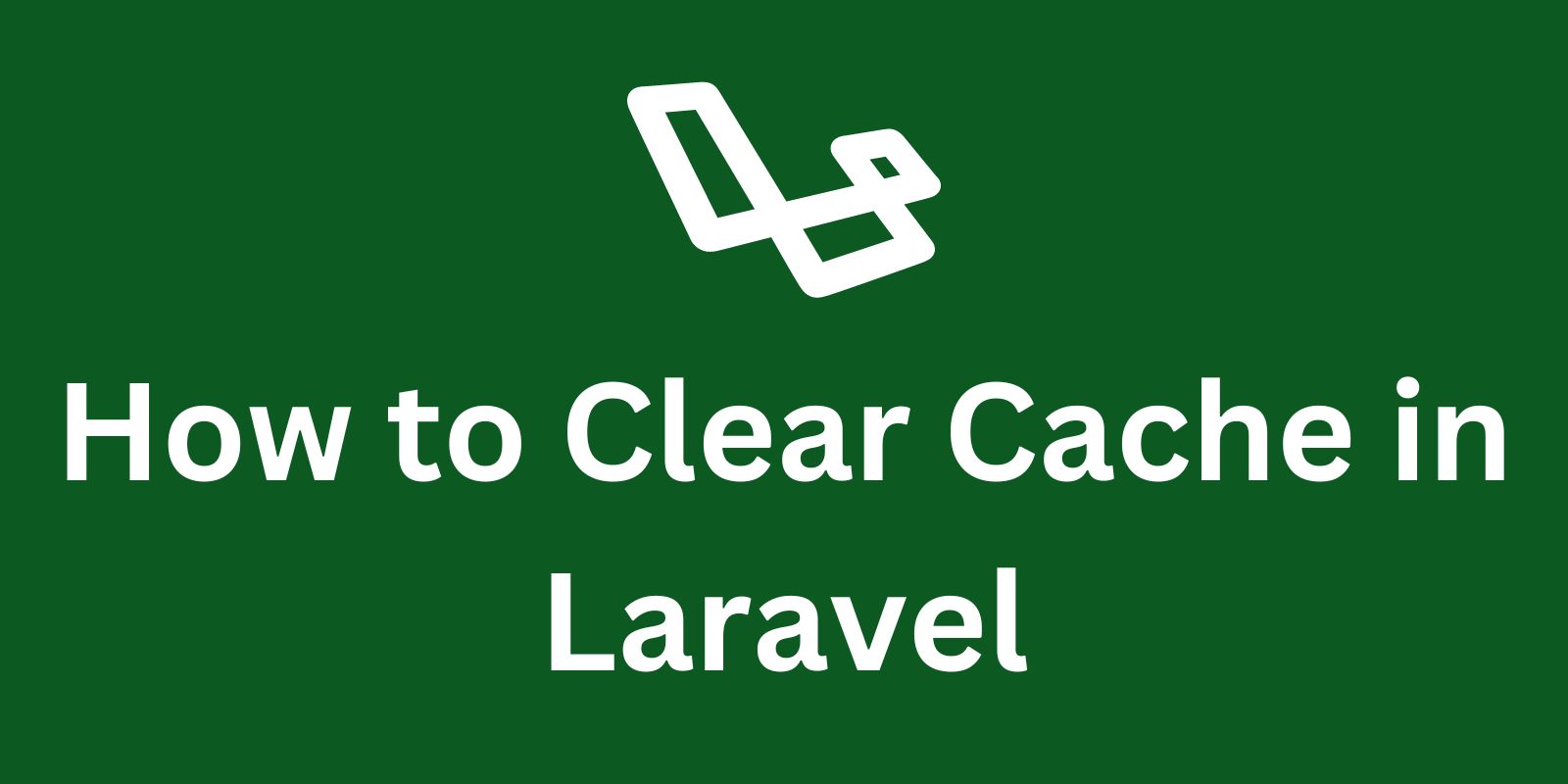
Caching is a crucial aspect of web development that improves performance by storing frequently accessed data. Laravel as a popular PHP framework provides powerful and robust caching mechanisms.
However, there are sometimes when you need to clear the cache to reflect the latest changes in your application. In this comprehensive guide, we’ll explore various methods to effectively clear Laravel’s cache.
To clear the cache in Laravel, you can use the PHP Artisan command-line interface and run ‘ php artisan cache:clear ’. This command removes all items from the cache, regardless of the cache driver being used. There are multiple other methods for clearing cache in Laravel and we are going to explore all of them in this guide.
Prerequisites:
- Basic understanding of the Laravel Framework
- Laravel installed on your machine
Table Of Contents
Understanding Laravel Cache
Before diving into clearing cache, let’s briefly understand how caching works in Laravel. Laravel provides support for various cache drivers like redis, Memcached and file-based caching. Cached data can include configurations, routes, views and more.
Why Clear Cache
To ensure that your application reflects the latest changes and avoids any unexpected behaviour caused by outdated cached data, Clearing cache becomes necessary when you make changes to your application that affect the cached data.
Methods to Clear Cache in Laravel
# Clearing Configuration Cache
Run the php artisan command ‘ php artisan config:clear ’ to clear the configuration cache. If you want to cache the latest configuration settings right away, you can run ‘ php artisan config:cache ’
php artisan config:clear
php artisan config:cache
# Clearing Route Cache
Run the php artisan command ‘ php artisan route:clear ’ to clear the route cache, ensuring that any changes made to your routes are reflected in your application.
php artisan route:clear
# Clearing View Cache
Run ‘ php artisan view:clear ’ to clear Laravel’s view cache. This command removes all the compiled view files, allowing Laravel to recompile them as required.
php artisan view:clear
# Clearing Application Cache
To clear all cached data in your application, including configurations, routes, views and more, run “ php artisan cache:clear ” artisan command. This command flushes the entire application cache.
php artisan cache:clear
# Using the ‘Cache’ facade
You can choose to flush all the application’s cached data using Laravel's ‘ Cache ’ facade.
use Illuminate\Support\Facades\Cache;
Cache::flush();
You can also use the ‘ Cache ’ facade to remove a specific item from the cache by passing its key to the ‘ forget() ’ method of the ‘ Cache ’ facade.
use Illuminate\Support\Facades\Cache;
Cache::forget('key');
#Using the ‘cache()’ helper function
You can also choose to use the ‘ cache() ’ helper function to both remove a specific item from the cache and as well as flushing the entire application cache.
cache()->forget('key');
cache()->flush();
# Clearing scheduled tasks cache
Run the artisan command ‘ php artisan schedule:clear-cache ’ to clear Laravel's scheduled tasks cache. Though it is strongly advised no to run this command in production, so proceed with caution.
php artisan schedule:clear-cache
# Turning off laravel application Cache
You can simply turn off the application caching mechanism if you need to by changing the ‘ CACHE_DRIVER ’ environment variable to null .
CACHE_DRIVER=null
Conclusion
We have explored various methods to clear cache in Laravel, ensuring that your application reflects the latest changes. Understanding when and how to clear Laravel’s cache helps you maintain optimal performance in your Laravel application and avoid issues caused by outdated cached data. By following the methods outlined in this guide, you can effectively clear cache in Laravel and maintain optimal performance for your Laravel applications.
Comment
Login to comment