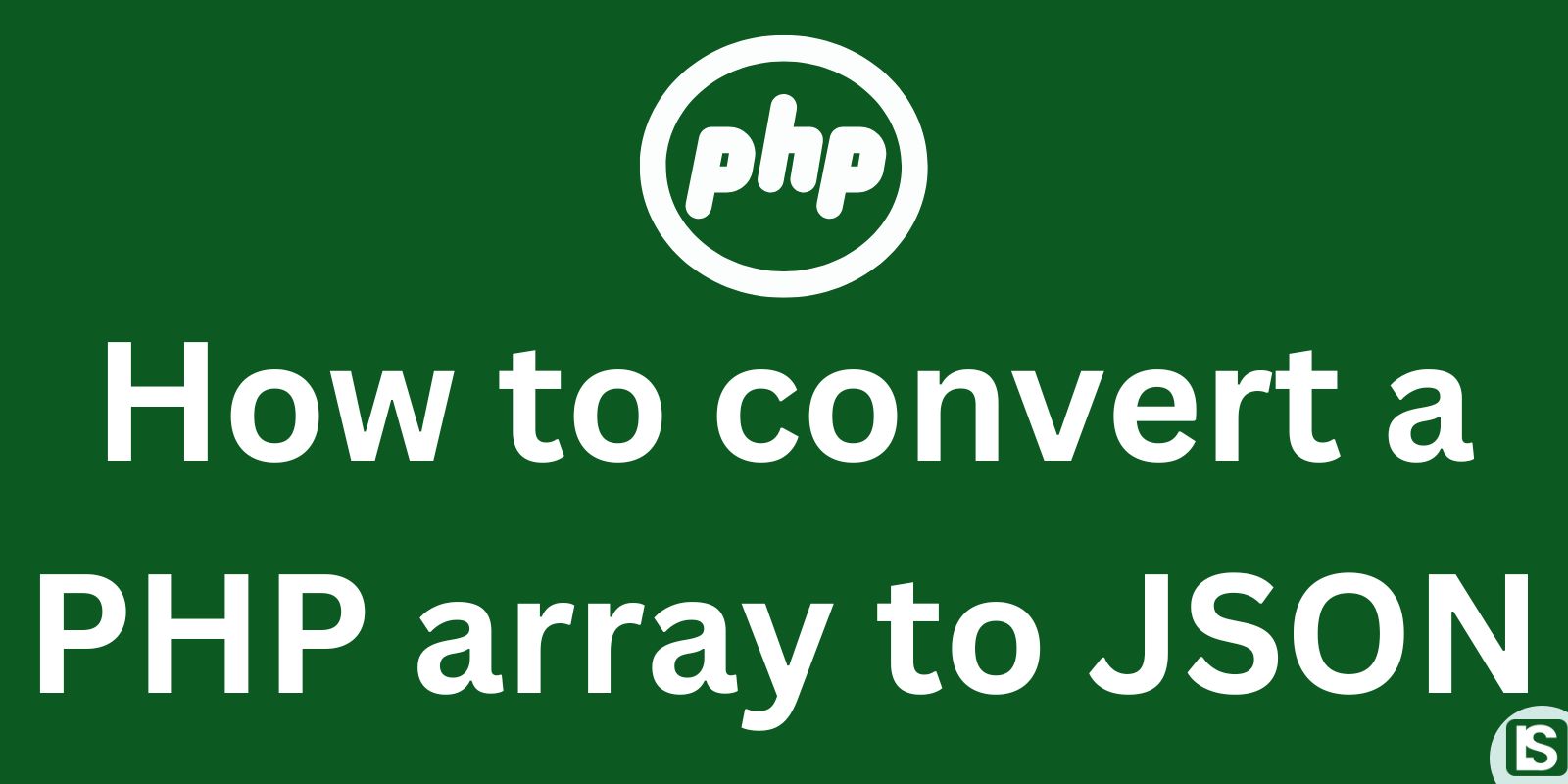
Table Of Contents
Introduction
In PHP coding, the need for data conversion to JSON format arises frequently, whether for saving data in files like log files, transmitting within a POST request to an external API, or for validating data format through JSON string printing. This comprehensive guide empowers you with essential know-how to effortlessly convert PHP arrays to JSON.
Convert PHP array to JSON
To convert a php array to json, we utilise the json_encode() function which is an inbuilt PHP function.
$car = ["name" => 'bmw', "year" => '2028', 'country' => 'baskon'];
$cars_json = json_encode($car);
echo $cars_json;
The above code prints a Json string containing the array elements in json format as shown below.
{"name":"bmw","year":"2028","country":"baskon"}
Convert JSON array to a PHP array
We can also convert the json data to a php array using the json_decode() function.
json_cars = '["jeep", "ford", "benz", "bmw"]';
$cars_array = json_decode($json_cars);
var_dump($cars_array); // array(4) {[0]=>string(4) "jeep"[1]=>string(4) "ford"[2]=>string(4) "benz"[3]=>string(3) "bmw"}
Conclusion
Different PHP data types, including arrays, objects, and strings, can be seamlessly converted to and from JSON format using the json_encode() and json_decode() functions, respectively. For instance, let's delve into the process of converting a standard PHP object to and from JSON format.
Create a php object.
Here we are going to define a class and create an object using that class
class Car {
public $name;
public $year;
public $price;
public function __construct($name, $year, $price){
$this->name = $name;
$this->year = $year;
$this->price = $price;
}
}
$jeep = new Car('jeep', '2020', 80000);
Convert php object to json
Now lets convert the $jeep object from the above snippet to JSON.
$json_jeep = json_encode($jeep);
echo $json_jeep; // {"name":"jeep","year":"2020","price":80000}
Convert json to a standard php object
Now lets convert the json data from the above snippet back to a php object
$std_jeep = json_decode($json_jeep);
echo ($std_jeep->price); // 80000
Comment
Login to comment