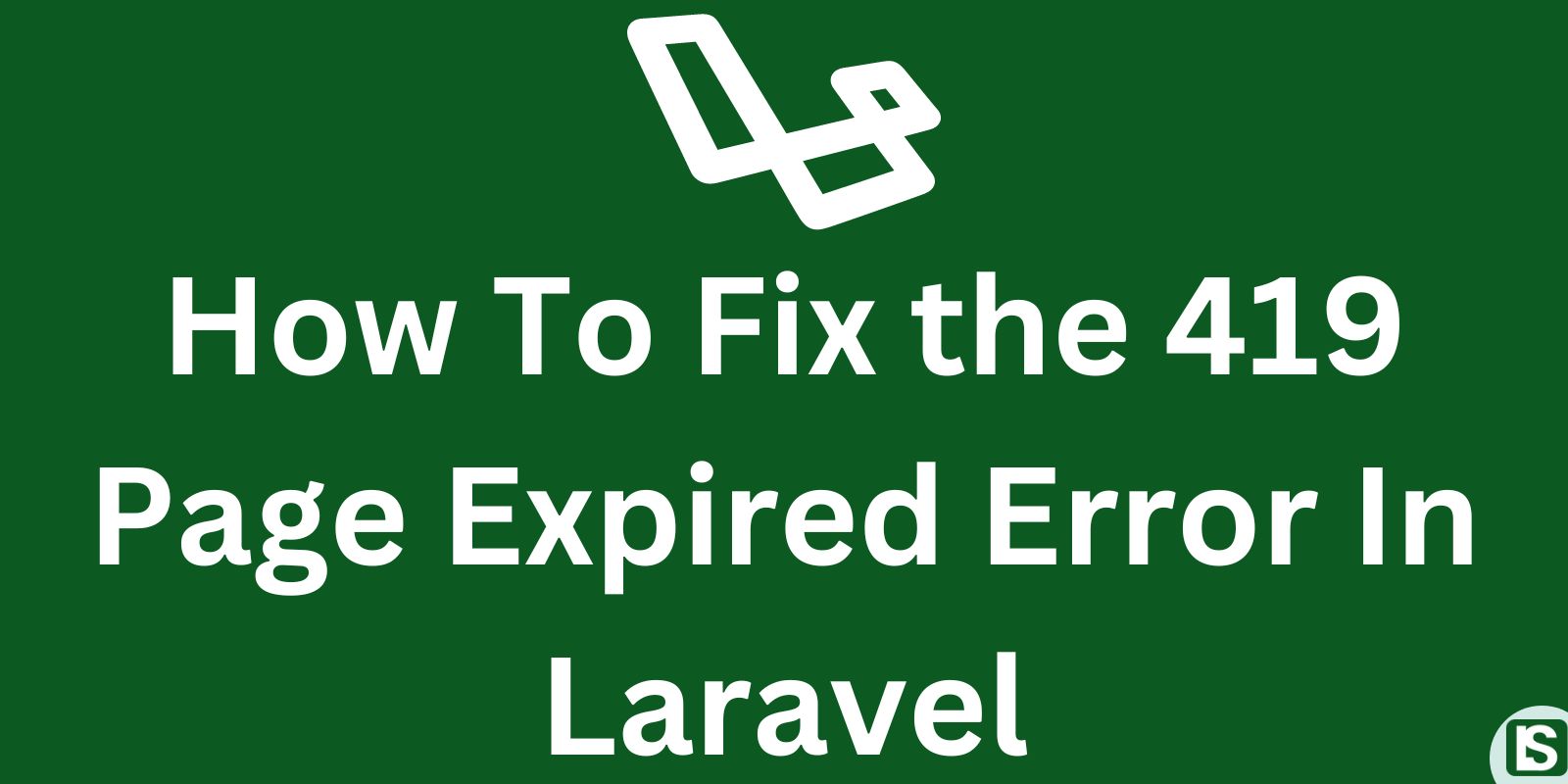
Table Of Contents
- Introduction
- Understanding the 419 Page Expired Error
- Common Causes of the 419 Error
- How To Fix the 419 Page Expired Error
- Conclusion
- Additional Resources
Introduction
Laravel provides robust features for web development. However, encountering errors like the “ 419 page expired ” error can be very frustrating for both the developers and the users. In this article, we will delve into the causes of this error and provide practical solutions to resolve it.
Understanding the 419 Page Expired Error
The “ 419 Page Expired ” error is very common in Laravel applications, typically triggered by session timeout or a CSRF (Cross-Site Request Forgery) token mismatch.
Laravel includes CSRF protection to prevent unauthorised requests, and this error occurs when the CSRF token validation fails.
Common Causes of the 419 Error
Session Timeout
Laravel has a default session lifetime configuration, which when exceeded, leads to session expiration and triggers the 419 error.
CSRF Token Mismatch
CSRF tokens are used to verify that the authenticated user is the one making the requests as well as making sure that the request is being made from within the application and not from a third party.
A token mismatch often caused by expired tokens or the absence of the _token CSRF token field in the submitted form results in the 419 error.
How To Fix the 419 Page Expired Error
Increasing Session Lifetime
To prevent premature session expiration, adjust the session lifetime configuration in the ‘ config/session.php ’ file. Increase the ‘ lifetime ’ value to extend the session duration.
'lifetime' => env('SESSION_LIFETIME', 120),
Refreshing the CSRF Token
Ensure that CSRF tokens are refreshed properly in Laravel forms. Use the ‘ @csrf ’ directive to include the CSRF token in your forms. The directive automatically adds the _token hidden field in the form which contains the CSRF token required for verification.
The token can expire when a user leaves the webpage dormant for too long and in this case the user just needs to refresh the page in order to have the CSRF token refreshed.
Handling Ajax Requests.
When making Ajax requests, ensure that CSRF tokens are included in the request headers or payload.
You can include the CSRF token in a meta tag.
<meta name="csrf-token" content="{{ csrf_token() }}">
Then you can easily instruct a library like jQuery to automatically add the token to all request headers. This provides simple, convenient CSRF protection for your AJAX based applications using legacy JavaScript technology.
$.ajaxSetup({
headers: {
'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content')
}
});
Disabling CSRF Protection On Some Pages
You can place the routes that you want to exclude from the CSRF protection outside of the ‘ routes/web.php ’.
You can also exclude the routes by adding their URIs to the ‘ $except ’ property of the ‘ verifyCsrfToken ’ middleware.
namespace App\Http\Middleware;
use Illuminate\Foundation\Http\Middleware\VerifyCsrfToken as Middleware;
class VerifyCsrfToken extends Middleware
{
/**
* The URIs that should be excluded from CSRF verification.
*
* @var array
*/
protected $except = [
'http://example.com/foo/bar',
'http://example.com/foo/*',
];
}
Conclusion
The ‘419 Page Expired’ error in Laravel can be resolved by addressing session timeout and CSRF token issues effectively. By understanding the causes of this error and following the step-by-step solutions provided in this guide, developers can ensure a seamless user experience in their Laravel application.
Comment
Login to comment