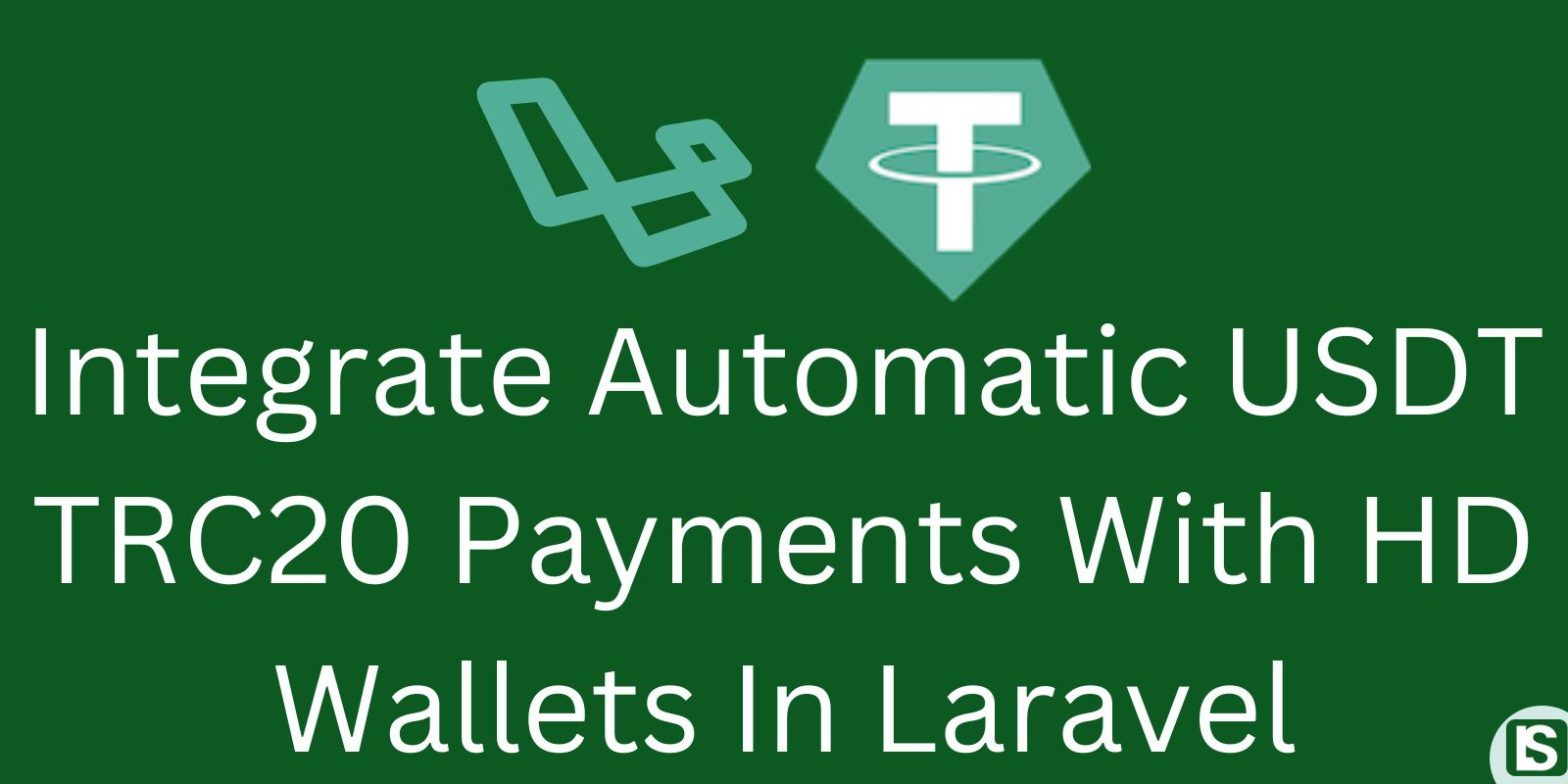
Are you running a Laravel application that involves processing user payments through donations or subscriptions? Whether you manage a Laravel-based online shopping website or aim to integrate USDT TRC20 payments, we've got you covered!
Explore our comprehensive step-by-step guide detailing the seamless integration of USDT TRC20 payments with HD Wallets into your Laravel application.
Table Of Contents
- Introduction
- Hierarchical Deterministic Wallets (HD) Wallets
- Energy Delegation
- Prerequisites
- Step 1: Install required Packages
- Step 2: Configuration
- Step 3: Testing and Running
Introduction
USDT TRC20 is a variant of the Tether (USDT) stable coin uniquely issued on the TRON blockchain, designed in accordance with the TRC20 token standard.
At present, USDT TRC20 stands as one of the most extensively utilized stable coins in online transactions and transfers, boasting an impressive daily trading volume exceeding $10 billion on average.
This guide serves as a clear and concise roadmap for seamlessly integrating USDT TRC20 payments into your Laravel website, allowing you to receive funds directly into your crypto wallet. By eliminating the reliance on third-party payment gateway services, this underscores the empowerment of decentralized finance.
Hierarchical Deterministic Wallets (HD) Wallets
Hierarchical Deterministic wallets use a hierarchical structure and a deterministic algorithm to generate an unlimited number wallet addresses from a single mnemonic phrase. To utilize this script, you just have to copy get the pnemonic phrase of your wallet preferably the Tronlink wallet and that is all you need for a wallet to start accepting automatic USDT TRC20 payments.
Energy Delegation
You can utilize the energy delegation functionality by simply having enough energy to process your daily transactions in the central wallet address which is the address at index zero of your Mnemonic phrase.
For energy aquisition, you can simply follow the steps for staking TRX for energy and bandwidth at the tronscan website or you can use any other preferred means of aquiring energy.
Prerequisites:
This guide assumes you are actively engaged in developing a Laravel application with user authentication and are keen to integrate USDT TRC20 payments, enabling seamless acceptance of USDT payments from your user base.
Additionally, it is assumed that you possess a Crypto wallet providing you with access to your wallet's Mnemonic Phrase.
Familiarity with the Laravel PHP framework is also presumed for a smoother understanding of the integration process.
With that said, let's dive in and get started!
Step 1: Install required Packages
To begin, you'll need to install the required PHP packages with Composer as shown bellow.
composer require bitwasp/bitcoin
composer require kornrunner/keccak
composer require simplito/elliptic-php
composer require simplesoftwareio/simple-qrcode "~4" --ignore-platform-reqs
Step 2: Configuration
As you already have your Laravel project in place, it's time to manage the configuration by adding the necessary models, controllers, and other essential files to the project.
Begin by downloading and extracting the USDT TRC20 Automatic Payments With HD Wallets for Laravel zipped file. The file structure is outlined below:
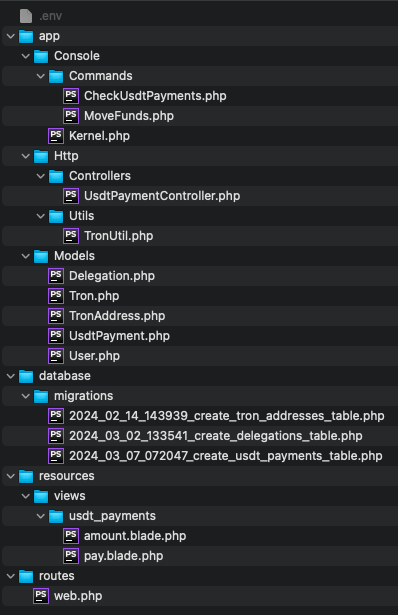
Now, proceed to copy the files into your Laravel application, following the designated file structure.
For certain files (app/Console/Kernel.php, app/Models/User.php, routes/web.php), simply copy the specified lines of code into the corresponding files in your Laravel application.
Copy files
To begin, copy the scheduler commands to your Laravel project.
app/Console/Commands/CheckUsdtPayments.php
app/Console/Commands/MoveFunds.php
Copy the controller into your project.
app/Http/Controllers/UsdtPaymentController.php
Copy the TronUtil.php file
app/Http/Utils/TronUtil.php
Copy the models to your project.
app/Models/Tron.php,
app/Models/UsdtPayment.php,
app/Models/TronAddress.php
app/Models/Delegation.php
Copy the migration file into your migrations folder.
database/migrations/2024_02_14_143939_create_tron_addresses_table.php,
database/migrations/2024_03_02_133541_create_delegations_table.php,
database/migrations/2024_03_07_072047_create_usdt_payments_table.php
Also copy the amount.blade.php and pay.blade.php files along with their directory to your views folder
usdt_payments/amount.blade.php,
usd_payments/pay.blade.php
Copy and paste code
app/Console/Kernel.php
Copy the following code into your schedule() function.
$schedule->command('app:check-usdt-payments')->everyMinute()->withoutOverlapping();
$schedule->command('app:move-funds')->everyThreeMinutes()->withoutOverlapping();
The cron job
To facilitate payment processing and maintain constant interaction with the TRON blockchain, the system employs a cron job/task scheduling to execute the scheduled commands mentioned above. For comprehensive guidance on managing Laravel task scheduling and cron jobs, refer to the Laravel documentation at Laravel Scheduling
For testing purposes, initiate the schedules by executing the following Artisan command.
php artisan schedule:run
Alternatively, you can directly execute the commands using the Artisan commands below for the 'check-usdt-payments', and 'move-funds' command.
Php artisan app:check-usdt-payments
Php artisan app:move-funds
app/Models/User.php
Copy the following code into your User Model.
public function usdtPayments(){
return $this->hasMany(UsdtPayment::class);
}
public function getTotalUsdtPayments(){
return $this->usdtPayments()->where('status', '=', 'success')->sum('amount');
}
routes/web.php
Copy the following code to your routes/web.php file
Route::middleware([
'auth:sanctum',
config('jetstream.auth_session'),
'verified',
])->group(function () {
Route::get('/usdt-payment', [
\App\Http\Controllers\UsdtPaymentController::class,
'showPayment',
]);
Route::post('/usdt-payment', [
\App\Http\Controllers\UsdtPaymentController::class,
'initiatePayment',
]);
Route::get('/pay-usdt', [
\App\Http\Controllers\UsdtPaymentController::class,
'pay',
]);
Route::get('/check_status', [
\App\Http\Controllers\UsdtPaymentController::class,
'checkStatus',
]);
});
Run the migrations.
Now, proceed to execute the migrations to update and generate the necessary tables in the database.
During this process, new tables will be generated, specifically the 'tron_addresses', 'delegations' and 'usdt_payments' table."
Execute the migrations by running the following artisan command below.
php artisan migrate
.env
Add the MNEMONIC_PHRASE, TRONGRID_URI and the TRONGRID_API_KEY in your .env file. The mnemonic phrase is a 12 word phrase the for your wallet and from it, all the payment addresses will be generated including the central wallet which will be the address at index zero.
The TRONGRID_API_KEY is the api key from your trongrid.io account which is required when accessing the trongrid node.
MNEMONIC_PHRASE=""
TRONGRID_API_KEY=""
TRONGRID_URI="https://api.trongrid.io"
Step 3: Testing and Running
With everything set up, it's time to proceed to test the payments.
Now, log in as a user and visit /usdt-payment. You should encounter a straightforward page prompting you to enter the desired payment amount before proceeding.
Feel free to customize the user interface and flow to align with the specific requirements of your application.
usdt_payments/amount.blade.php
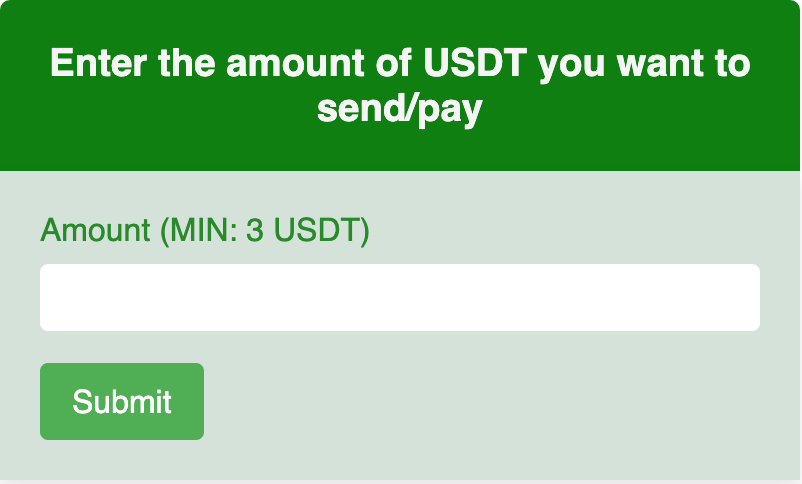
Next, you will be redirected to a page displaying the payment address and QR code.
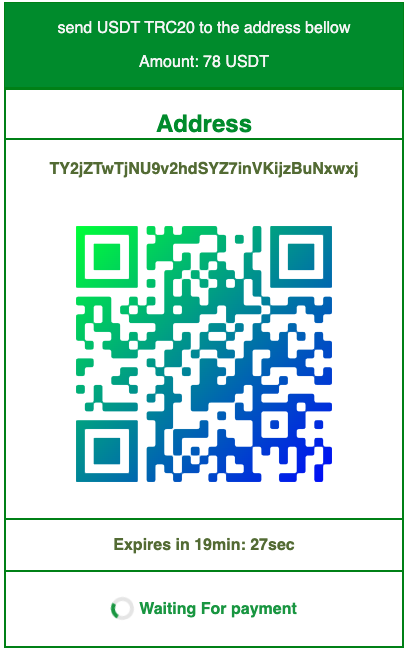
After making the payment to the displayed wallet address, execute the following Artisan commands to process the payment by interacting with the TRON blockchain in the development environment
Php artisan app:check-usdt-payments
Php artisan app:move-funds
After sending the funds to the specified address, You should receive a successful alert indicating that the payment was processed successfully.
With all configurations in place, the funds should be seamlessly transferred to your central wallet.
With that said, you should now be equipped to proceed and make the final adjustments in accordance with your specific application requirements.
Comment
Login to comment