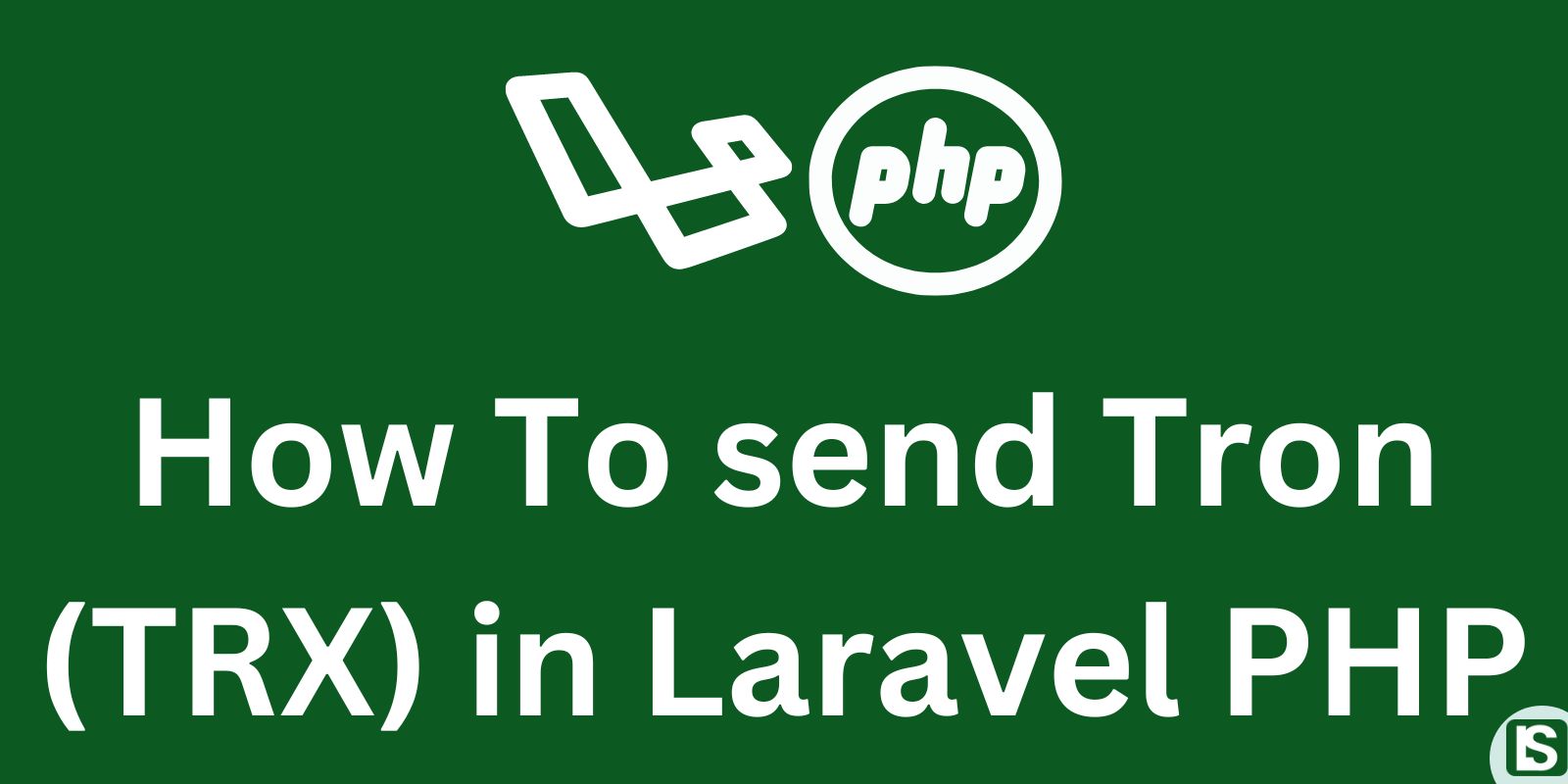
Table of Contents
Introduction
In this article, you'll discover the process of transferring Tron (TRX) from one address to another in Laravel PHP.To initiate the transfer, we'll begin by creating a send transaction, signing it with the sender's private key, and finally broadcasting it to the blockchain network.
Prerequisites
We'll leverage the Guzzle HTTP package, which comes pre-installed with Laravel. Additionally, you'll require a Trongrid API key for connecting to the Tron Mainnet, obtainable from the Trongrid website .
Send Tron (TRX) with Laravel PHP
<?php
namespace App\Http\Controllers;
use GuzzleHttp\Client;
use Illuminate\Http\Request;
class TronController extends Controller
{
public function broadcastTransaction($transaction){
$client = new Client();
$body = json_encode($transaction);
$response = $client->request('POST', 'https://api.trongrid.io/wallet/broadcasttransaction', [
'body' => $body,
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
'TRON-PRO-API-KEY' => 'Your Trongrid api key'
],
]);
return json_decode($response->getBody());
}
public function signTransaction($transaction_id, $private_key){
// Get the transaction signing algorithm for just $20 at https://lakescripts.com/shop/php-tron-signer---sign-tron-blockchain-transactions-in-your-php-application
}
public function sendTRX($amount, $sender_address, $sender_private_key, $receiver ){
//convert trx to sun
$amount_sun = $amount * 1000000;
$body = "{\"owner_address\":\"$sender_address\",\"to_address\":\"$receiver\",\"amount\":$amount_sun,\"visible\":true}";
//initiate guzzle client
$client = new Client();
$response = $client->request('POST', 'https://api.trongrid.io/wallet/createtransaction', [
'body' => $body,
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
'TRON-PRO-API-KEY' => 'Your Trongrid api key'
],
]);
$txn = json_decode($response->getBody());
if(isset($txn->txID) && $txn->txID != null){
$signature = $this->signTransaction($txn->txID, $sender_private_key);
$txn->signature = [$signature];
$transaction = $this->broadcastTransaction($txn);
if($transaction->result && isset($transaction->txid) && $transaction->txid != null){
return $transaction->txid; //return the transaction hash
}else{
return false;
}
}else{
return false;
}
}
}
Initiate Transaction
As illustrated in the code snippet above, sending TRX is simplified to calling the sendTRX() method within our TronController, passing it four parameters, namely:
- $amount : The amount of trx to send.
- $sender_address : The wallet address of the sender,
- $sender_private_key : The private key of the sender’s wallet,
- $receiver : The receiver’s wallet address.
The sendTRX() function triggers the transaction initiation process by sending an HTTP POST request to Trongrid's 'wallet/createtransaction' endpoint. This request includes parameters such as the sender address, receiver address, and the amount in SUN. In response, the endpoint provides the initiated transaction data in JSON format.
$amount_sun = $amount * 1000000;
$body = "{\"owner_address\":\"$sender_address\",\"to_address\":\"$receiver\",\"amount\":$amount_sun,\"visible\":true}";
//initiate guzzle client
$client = new Client();
$response = $client->request('POST', 'https://api.trongrid.io/wallet/createtransaction', [
'body' => $body,
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
'TRON-PRO-API-KEY' => 'Your Trongrid api key'
],
]);
$txn = json_decode($response->getBody());
Sign Transaction.
Now that we possess the initiated transaction data, it's time to sign the transaction using the sender's private key. As depicted in the snippet above, we extract the transaction ID from the transaction data and invoke the signTransaction() function. This function accepts the transaction ID and the sender's private key as parameters, returning the transaction signature.
$signature = $this->signTransaction($txn->txID, $sender_private_key);
PHP Tron Signer
Access the transaction signing logic for just $20 at PHP Tron Signer
Broadcast Transaction
The concluding step involves broadcasting the signed transaction to the Tron Mainnet. Upon obtaining the transaction signature, we incorporate it into our initiated transaction data, preparing it for broadcast across the network.
$txn->signature = [$signature];
$transaction = $this->broadcastTransaction($txn);
if($transaction->result && isset($transaction->txid) && $transaction->txid != null){
return $transaction->txid; //return the transaction hash
}else{
return false;
}
We proceed to invoke the broadcastTransaction() method with our transaction data. This action sends an HTTP POST request to Trongrid's 'wallet/broadcasttransaction' endpoint, effectively broadcasting the transaction to the network. Subsequently, we receive a response containing the broadcasted transaction data, from which we extract the transaction hash.
Conclusion
You've mastered the steps to initiate, sign, and broadcast Tron (TRX) transfer transactions on the Tron blockchain network. With this knowledge, you're equipped to seamlessly integrate Tron transfers into your PHP applications.
Comment
Login to comment