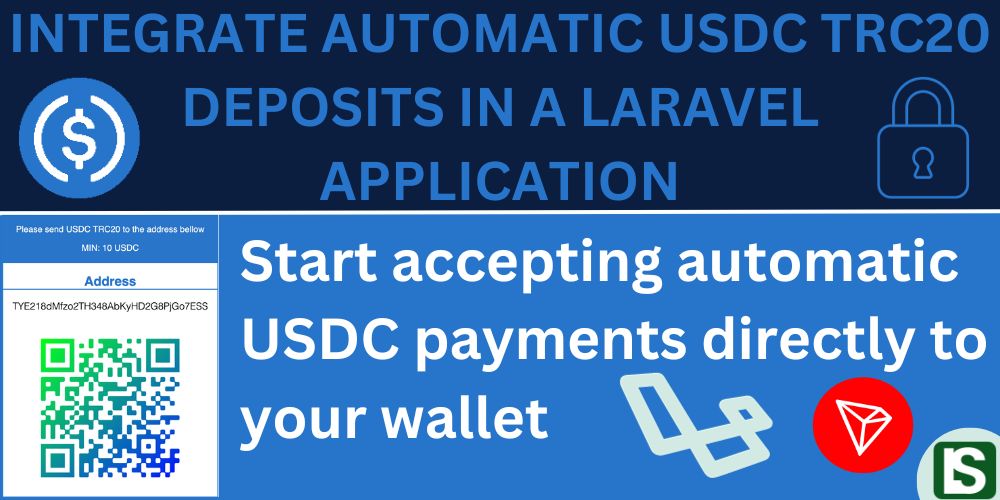
Are you working on a fresh laravel application or has one already running and you want to integrate USDC TRC20 deposits? Here is a step by step guide to help you start accepting automatic USDC TRC20 deposits in your laravel website.
Introduction:
USDC TRC20 is a version of the USDC stable coin issued on the TRON blockchain adhering to the TRC20 token standard.
Integrate automatic USDC deposits in your website to appreciate the true potential of crypto and decentralized finance. With instant fund transfers directly into your wallet without ever having to worry about dependency on financial intermediaries like banks and other payment gateways, full financial control over your online business is always in your grip.
Prerequisites:
This documentation expects you to be working with a laravel application that has user authentication and you are looking to integrate automatic USDC TRC20 deposits for the users.
You are also expected to have a tron wallet preferably the Tronlink wallet that allows you to export your private key.
Step 1: Install required Packages
For the start, you need to install the required laravel packages and in this case we need specifically two packages ie;
IEXBase/tron-api
This is a php package for interacting with the tron blockchain.
composer require iexbase/tron-api --ignore-platform-reqs
simplesoftwareio/simple-qrcode
This is a php package for generating qr codes which make it easier for the user to simply scan the qr code for the deposit wallet address from their mobile device.
composer require simplesoftwareio/simple-qrcode "~4" --ignore-platform-reqs
Step 2: Set Up Tronlink Wallet
If you currently do not have the tronlink wallet app or extension you need to install it from tronlink.org and create a tron wallet.
Step 3: Configuration
Since you already have the laravel project by now, it's time to handle the configuration to add the required models, controller and other required files in the project.
First you need to download and unzip the USDC TRC20 AUTOMATIC DEPOSITS FOR LARAVEL zipped file and its file structure is as shown below.
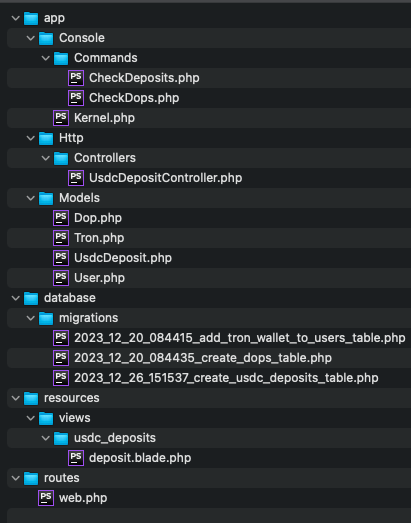
Now you have to copy the files into your laravel application in accordance with the file structure.
For some files (app/Console/Kernel.php, app/Models/User.php, routes/web.php) you just have to copy the specified lines of code into the respective files in your laravel application.
Copy files
First, you have to copy the commands to your laravel project
app/Console/Commands/CheckDeposits.php,
app/Console/Commands/CheckDops.php
Copy the controller to your project
app/Http/Controllers/UsdcDepositController.php
Copy the three models to your project.
app/Models/Dop.php,
app/Models/Tron.php,
app/Models/UsdcDeposit.php
Copy the migration files into your migrations folder.
database/migrations/2023_12_20_084415_add_tron_wallet_to_users_table.php
database/migrations/2023_12_20_084435_create_dops_table.php
database/migrations/2023_12_26_151537_create_usdc_deposits_table.php
Also copy the deposit.blade file along with its directory to your views folder.
usdc_deposits/deposit.blade.php
Copy and paste code
app/Console/Kernel.php
Copy the following code into your schedule() function.
$schedule->command('app:check-dops')->everyTenMinutes()->withoutOverlapping();
$schedule->command('app:check-deposits')->everyThreeMinutes()->withoutOverlapping();
The cron job
To process the deposits and continuously interact with the tron blockchain, the system uses a cron job / task scheduling to run the above scheduled commands. For details on how to handle laravel task scheduling and cron job, check out the laravel docs at Laravel Scheduling
For the purpose of testing can run the schedules buy running the following artisan commands
php artisan schedule:run
Or you can run the commands directly with the artisan commands below for the check-dops command and check-deposits command.
php artisan app:check-dops
php artisan app:check-deposits
app/Models/User.php
Copy the following code into your User Model.
public function dops(){
return $this->hasMany(Dop::class);
}
public function usdcDeposits(){
return $this->hasMany(UsdcDeposit::class);
}
public function getTotalUsdcDeposits(){
return $this->usdcDeposits()->sum('amount');
}
routes/web.php
Copy the following code to your routes/web.php file
Route::middleware([
'auth:sanctum',
config('jetstream.auth_session'),
'verified',
])->group(function () {
Route::get('/usdc-deposit', [\App\Http\Controllers\UsdcDepositController::class, 'deposit']);
});
.env file
You now need to add the following in the env file to set the required minimum deposit amount.
MIN_USDC_DEPOSIT=10
Run the migrations.
It is now time to run the migrations so that they can update and generate the required tables in the database.
Two new tables will be generated ie; dops and usdc_deposits table.
The users table will be updated to add two columns (tron_wallet_address, tron_wallet_key).
Execute the migrations by running the following artisan command below.
php artisan migrate
Export tron wallet address and private key.
The final configuration is to export your tron wallet address and the tron wallet private key and these are going to be added to the system specifically in the Tron.php file.
For a detailed guide on how to export the tron private key, check out my article on How To Export Tron Private Key From Tronlink Wallet
app/Models/Tron.php
Your tron wallet is going to handle specifically two purposes ie;
- First, it is going to be your central wallet that receives all the funds deposited by the users.
- Second, it is going to be providing fees in the form of TRX to be used in the transfer of the USDT TRC20 tokens to the central wallet (itself). So make sure to atleast have a minimum balance of 100TRX in the wallet.
Now that you have exported the address and the private key, you are going to fill them in the Tron.php model (app/Models/Tron.php) as directed bellow
private $deposit_wallet = "wallet address";
private $deposit_key = "wallet private key";
Step 4: Testing and Running
Now that you have everything set up, it is time to test the deposits.
You can now login and navigate to /usdc-deposit and you should get a simple deposit page with the payment address displayed and it should look like the image below. Feel free to customise the page to meet your ui requirements.
resources/views/usdc_deposits/deposit.blade.php
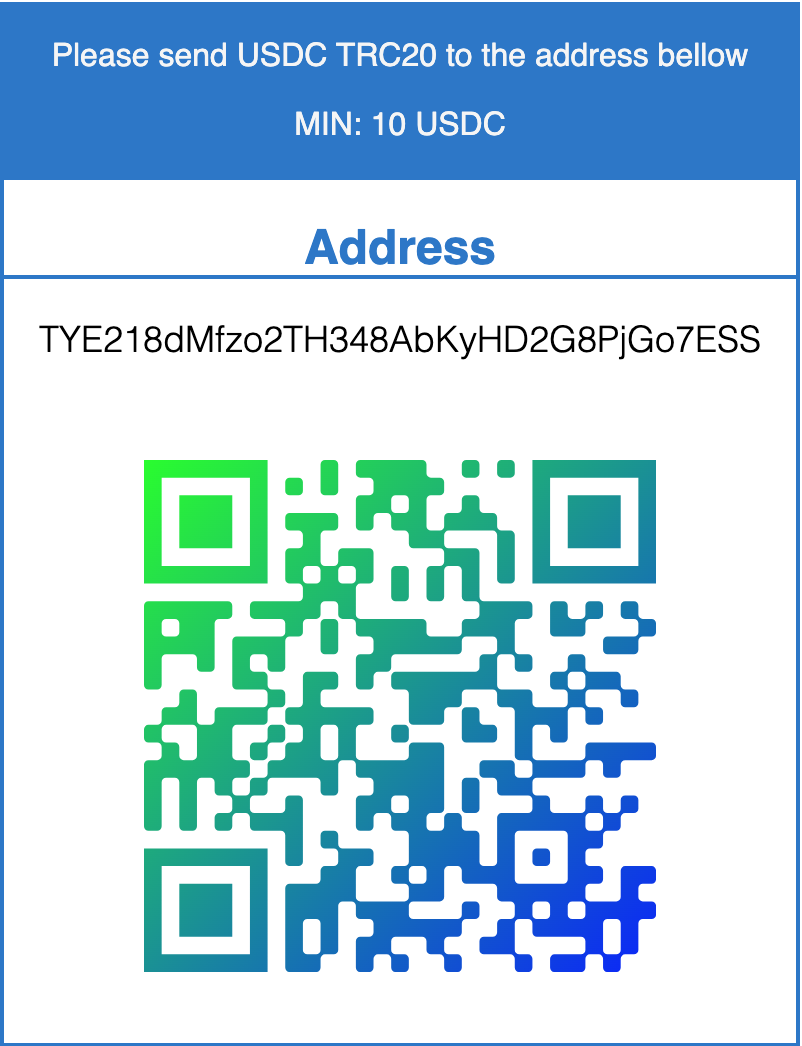
You can now make a deposit to that displayed wallet address and then run the artisan command below to have the deposit processed by interacting with the tron blockchain on the development environment.
php artisan app:check-deposits
Now the deposit should be processed and the details filled in the database.
With that said, now you should be able to take it from here and do the final touches such as updating user balances and so on.
Comment
Login to comment